This post explains how to use jQuery FlexBox plugin for Google like auto-suggestion box to edit data in jqGrid. Suppose jqGrid has Country column and we want to give auto-suggestion box during editing. When user enters letter, It will auto-populate related country names.
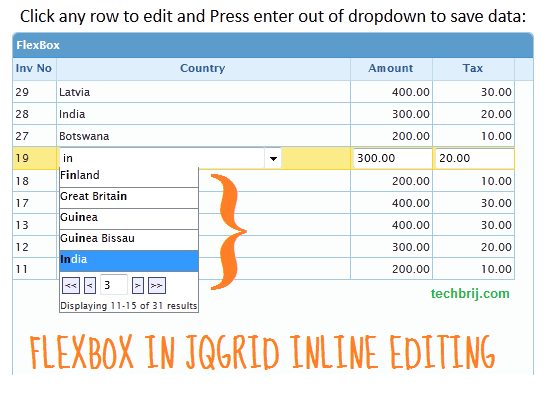
CSS & JS:
We have to include jquery UI, FlexBox and jqGrid css files.
<link rel="stylesheet" type="text/css" href="css/main.css" />
<link rel="stylesheet" type="text/css" href="css/jquery.flexbox.css" />
<link rel="stylesheet" type="text/css" href="css/jquery-ui-1.8.1.custom.css" />
<link rel="stylesheet" type="text/css" href="css/ui.jqgrid.css" />
<style type="text/css">
body{
text-align:center;
}
#gridMain td.countryHolder{
overflow:visible;
margin-bottom:20px;
}
#gridMain .ffb{
margin-top:22px;
}
.ui-jqgrid{
margin:0 auto;
}
</style>
Also, need to include jquery, jquery ui, FlexGrid, jqgrid locale and jqgrid js files.
<script type="text/javascript" src="js/jquery/1.4.2/jquery.min.js"></script>
<script type="text/javascript" src="js/jquery.flexbox.js"></script>
<script type="text/javascript" src="js/jquery-ui-1.8.20.min.js"></script>
<script type="text/javascript" src="js/grid.locale-en.js"></script>
<script type="text/javascript" src="js/jquery.jqGrid.min.js"></script>
<script type="text/javascript" src="js/countries.js"></script>
countries.js file has country data in following format:
var countries = {};
countries.results = [
{id:'AF',name:'Afghanistan'},
{id:'AL',name:'Albania'},
{id:'DZ',name:'Algeria'},
{id:'AS',name:'American Samoa'},
{id:'AD',name:'Andorra'},
{id:'AO',name:'Angola'},
{id:'AI',name:'Anguilla'},
{id:'AG',name:'Antigua & Barbuda'},
{id:'AR',name:'Argentina'},
{id:'AM',name:'Armenia'},
{id:'AW',name:'Aruba'},
{id:'AU',name:'Australia'},
{id:'AT',name:'Austria'},
{id:'AZ',name:'Azerbaijan'},
{id:'AP',name:'Azores'},
{id:'BS',name:'Bahamas'},
{id:'BH',name:'Bahrain'},
{id:'BD',name:'Bangladesh'},
.....
{id:'YE',name:'Yemen'},
{id:'ZM',name:'Zambia'},
{id:'ZW',name:'Zimbabwe'}
];
countries.total = countries.results.length;
See following for jqGrid data:
var mydata=[
{id:"11",Country:"Finland",amount:"200.00",tax:"10.00"},
{id:"12",Country:"Australia",amount:"300.00",tax:"20.00"},
{id:"13",Country:"Bangladesh",amount:"400.00",tax:"30.00"},
{id:"27",Country:"Botswana",amount:"200.00",tax:"10.00"},
{id:"19",Country:"Liberia",amount:"300.00",tax:"20.00"},
{id:"17",Country:"Belgium",amount:"400.00",tax:"30.00"},
{id:"18",Country:"Belarus",amount:"200.00",tax:"10.00"},
{id:"28",Country:"India",amount:"300.00",tax:"20.00"},
{id:"29",Country:"Latvia",amount:"400.00",tax:"30.00"}];
HTML Structure:
<table id="gridMain"></table>
<div id="pagernav"></div>
<div id="testData" style="margin:0 auto"></div>
<input type="button" id="btnGetData" value="Get Updated Info" />
testData div is used to retrieve the latest data from jqGrid.
Read Also:
jqGrid: Drag and Drop columns (Reordering) with Javascript Array
jqGrid:
var lastsel2;
jQuery("#gridMain").jqGrid({
datatype: "local",
data: mydata,
pager: '#pagernav',
sortname: 'id',
sortorder: "desc",
sortable: true,
height: 300,
localReader : {id:'id'},
editurl: 'clientArray',
onSelectRow: function(id){
if(id && id!==lastsel2){
jQuery('#gridMain').restoreRow(lastsel2);
jQuery('#gridMain').editRow(id,true);
lastsel2=id;
}
},
colNames: ['Inv No', 'Country', 'Amount', 'Tax'],
colModel:[ {name:'id',index:'id',key: true, width:40},
{name:'Country',index:'Country', width:300, classes:"countryHolder", editable: true
,edittype:'custom',editoptions:{custom_element:myelem,custom_value:myval}
},
{name:'amount',index:'amount', width:80, align:"right", editable: true},
{name:'tax',index:'tax', width:80, align:"right", editable: true}
],
caption: "FlexBox"
});
jQuery("#gridMain").jqGrid('navGrid', '#pagernav', {add:false,edit:false,del:false});
We defined country colum edittype = custom and defined myelem to get custom control and myval to get value in editoptions. In myelem method, FlexBox is configured.
function myelem(value,options){
var $ret = $('<div id="country"></div>');
$ret.flexbox(countries, {
initialValue: value,
paging: {
pageSize: 5
}
});
return $ret;
}
function myval(elem){
return $('#country_input').val();
}
You can configure more as per your requirement, check following:
Practical Usage:
For simplicity, I took local data. You can consume web service to get JSON data for grid and auto-complete list, modify grid data and pass to web service to save it. If you want to get the latest data from the grid, see following:
$("#btnGetData").click(function ()
{
var latestData = $('#gridMain').jqGrid('getRowData');
var record,tbl = '<table style="border:2px solid gray;margin:0 auto" cellspacing="0" cellpadding="3" >';
for (row in latestData){
record = latestData[row];
tbl += '<tr>';
for (cell in record)
{
tbl += '<td style="border:1px solid gray"> ' +record[cell] + '</td>';
}
tbl += '</tr>';
}
tbl += '</table>';
$("#testData").html(tbl);
});
Hope, It helps.