This post explains how you can display your featured posts using content slider to save page space and to attract visitors using jQuery and ASP.NET Web API. I am following this tutorial which explains how to implement with jQuery UI but it is static. We'll dynamically generate content slider and give sliding effect for image caption.
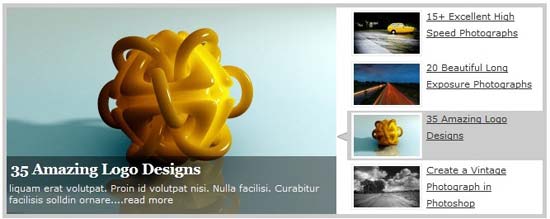
Web API:
You might want to get Admin selected posts, images or content from database and display them as content slider. So here web API's responsibility is to provide data.
Consider following Model:
public class Banner {
public string Title { get; set; }
public string URL { get; set; }
public string Summary { get; set; }
public string SmallImageURL { get; set; }
public string FullImageURL { get; set; }
}
In ValuesController, Get Method will return collection of banner objects. For simplicity, the data is hard-coded, but you can get from database and return in same way.
public IEnumerable<Banner> Get()
{
return new Banner[] {
new Banner(){ Title="15+ Excellent High Speed Photographs",
URL ="https://techbrij.com",
Summary="Lorem ipsum dolor sit amet, consectetur adipiscing elit. Nulla tincidunt condimentum lacus. Pellentesque ut diam....",
SmallImageURL ="images/Banner/image1-small.jpg",
FullImageURL="images/Banner/image1.jpg" },
new Banner(){ Title="20 Beautiful Long Exposure Photographs",
URL ="https://techbrij.com",
Summary="Vestibulum leo quam, accumsan nec porttitor a, euismod ac tortor. Sed ipsum lorem, sagittis non egestas id, suscipit....",
SmallImageURL ="images/Banner/image2-small.jpg",
FullImageURL="images/Banner/image2.jpg" },
new Banner(){ Title="35 Amazing Logo Designs",
URL ="https://techbrij.com",
Summary="liquam erat volutpat. Proin id volutpat nisi. Nulla facilisi. Curabitur facilisis sollicitudin ornare....",
SmallImageURL ="images/Banner/image3-small.jpg",
FullImageURL="images/Banner/image3.jpg" },
new Banner(){ Title="Create a Vintage Photograph in Photoshop",
URL ="https://techbrij.com",
Summary="Quisque sed orci ut lacus viverra interdum ornare sed est. Donec porta, erat eu pretium luctus, leo augue sodales....",
SmallImageURL ="images/Banner/image4-small.jpg",
FullImageURL="images/Banner/image4.jpg" }
};
}
For testing, Open following URL in browser, you will get the data
http://myservername/api/values
See following data in firebug:
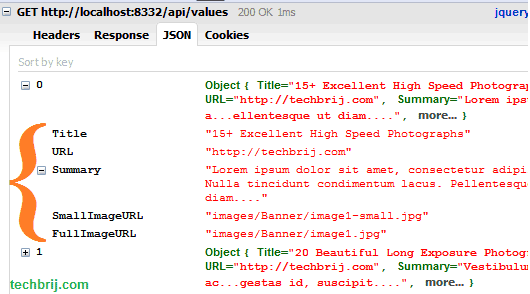
View:
For simplicity, we are taking same app, create new action in Home controller, add view without layout and add jQuery and jQuery UI in the view.
@Styles.Render( "~/Content/css")
@Scripts.Render("~/bundles/modernizr", "~/bundles/jquery", "~/bundles/jqueryui")
HTML Structure:
We are going to implement same structure as in this tutorial, but dynamically using jQuery. So in the view, we have following structure in body:
<div id="featured">
<ul class="ui-tabs-nav">
</ul>
</div>
That's it. To draw internal structure, I created following javascript method:
function drawLayout(banners) {
var temp;
$.each(banners, function (index, val) {
//Add Tab
$('#featured ul.ui-tabs-nav').append('<li class="ui-tabs-nav-item" id="nav-fragment-' + index + '"><a href="#fragment-' + index + '"><img src="../../' + val.SmallImageURL + '" alt="" /><span>' + val.Title + '</span></a></li>');
//Add Content
temp = [];
temp.push('<div id="fragment-' + index + '" class="ui-tabs-panel ui-tabs-hide">');
temp.push('<img src="../../' + val.FullImageURL + '" />');
temp.push('<div class="info" >');
temp.push('<h2><a href="' + val.URL + '" >' + val.Title + '</a></h2>');
temp.push('<p>' + val.Summary + '<a href="' + val.URL + '" >read more</a></p>');
temp.push('</div>');
temp.push('</div>');
$('#featured').append(temp.join(''));
});
}
To get data and add content dynamically, jquery ajax is used:
$.ajax({
url: '/api/values',
type: 'GET',
async: false,
dataType: 'json',
success: function (json) {
drawLayout(json);
},
error: function (XMLHttpRequest, textStatus, errorThrown) {
//alert('error - ' + textStatus);
console.log('error', textStatus, errorThrown);
}
});
To make content slider work:
//Show first slide by default
$('#featured .ui-tabs-panel:first').removeClass('ui-tabs-hide');
$('#featured .ui-tabs-panel:first .info').css({ top: '180px' });
$("#featured").tabs({ fx: { opacity: "toggle"} }).tabs("rotate", 5000, true);
It will create jQuery UI rotating tabs acts as auto rotating content slider. I want to display image caption animated when slide is displayed, so added following code:
$('#featured').bind('tabsshow', function (event, ui) {
var $ctrl = $("#featured .ui-tabs-panel:not(.ui-tabs-hide)").find('.info');
$ctrl.css({ top: '0px' });
$ctrl.stop().animate({ top: '180px' }, { queue: false, duration: 300 });
});
Check following HTML demo, instead of calling web api, just used javascript variable having JSON data.
Hope, It helps.