By Default, There is no RepeatColumns property in ASP.NET Gridview to display rows in multiple columns as in DataList control. When Gridview has few columns, It doesn't take full space of browser. To make good UI, You might need RepeatColumns property. This post explains how to display asp.net GridView rows in multiple columns using jQuery WITHOUT modifying any existing code.
Code:
function GridViewRepeatColumns(gridviewid, repeatColumns) {
//Created By: Brij Mohan(https://techbrij.com)
if (repeatColumns < 2) {
alert('Invalid repeatColumns value');
return;
}
var $gridview = $('#' + gridviewid);
var $newTable = $('<table></table>');
//Append first row in table
var $firstRow = $gridview.find('tr:eq(0)'),
firstRowHTML = $firstRow.html(),
colLength = $firstRow.children().length;
$newTable.append($firstRow);
//Append first row cells n times
for (var i = 0; i < repeatColumns - 1; i++) {
$newTable.find('tr:eq(0)').append(firstRowHTML);
}
while ($gridview.find('tr').length > 0) {
var $gridRow = $gridview.find('tr:eq(0)');
$newTable.append($gridRow);
for (var i = 0; i < repeatColumns - 1; i++) {
if ($gridview.find('tr').length > 0) {
$gridRow.append($gridview.find('tr:eq(0)').html());
$gridview.find('tr:eq(0)').remove();
}
else {
for (var j = 0; j < colLength; j++) {
$gridRow.append('<td></td>');
}
}
}
}
//update existing GridView
$gridview.html($newTable.html());
}
You have to use above method and pass gridviewid and number of repeated columns. suppose your gridview ID is gridSample and have following structure:
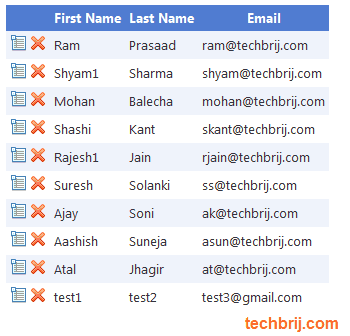
To create two columns layout, call following in javascript:
GridViewRepeatColumns("<%=gridSample.ClientID %>",2);
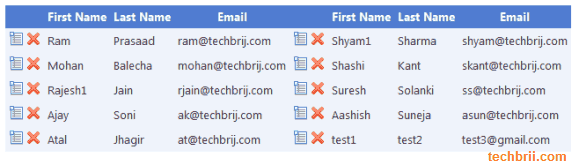
For Three columns layout:
GridViewRepeatColumns("<%=gridSample.ClientID %>",3);
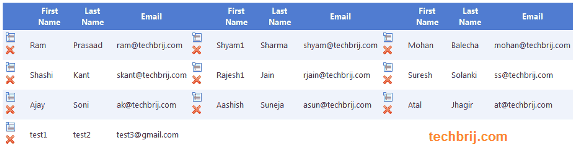
For Four columns layout:
GridViewRepeatColumns("<%=gridSample.ClientID %>",4);

Now You see edit and delete functionality is as it is, No need to modify any other server side code.
Demo:
Here is the HTML rendered demo of 3 columns layout:
Hope, It saves your time.