As the current trend is to make website compatible with all platforms like desktop, tablet and mobile..etc, Responsive Design technique comes into the picture. Using it, the website adapts the layout to the environment that it is being viewed in. In this post, we will make ASP.NET gridview responsive using jQuery FooTable plugin. It hides certain columns of data at different resolutions based on data-* attributes and converts hidden data to expandable rows. First, I recommend to read this post to understand how it works.
1. Download and add FooTable js, css and image files in the project and reference in the page.
<meta name="viewport" content = "width = device-width, initial-scale = 1.0, minimum-scale = 1.0, maximum-scale = 1.0, user-scalable = no" />
<link href="Styles/footable-0.1.css" rel="stylesheet" type="text/css" />
<script src="Scripts/1.8.2/jquery.min.js" type="text/javascript"></script>
<script src="Scripts/footable-0.1.js" type="text/javascript"></script>
Read Also: How to Test Responsive Web Design
GridView:
2. Here is the asp.net gridview structure, we take address as template column for testing:
<asp:GridView ID="GridView1" CssClass="footable" runat="server"
AutoGenerateColumns="False">
<Columns>
<asp:BoundField DataField="FirstName" HeaderText="First Name" />
<asp:BoundField DataField="LastName" HeaderText="Last Name" />
<asp:BoundField DataField="Email" HeaderText="Email" />
<asp:TemplateField HeaderText="Address">
<ItemTemplate>
<asp:Label ID="Label1" runat="server" Text='<%# Bind("Address") %>'></asp:Label>
</ItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="Contact" HeaderText="Contact" />
</Columns>
</asp:GridView>
Breakpoints:
3. This plugin works with the concepts of "breakpoints", which are different device widths we care about.
$(function () {
$('#<%=GridView1.ClientID %>').footable({
breakpoints: {
phone: 480,
tablet: 1024
}
});
});
4. To bind gridview:
GridView1.DataSource = GetDataTable();
GridView1.DataBind();
GetDataTable method returns data for gridview. You can implement it to get data from database and returns datatable or list.
By default asp.net gridview header row is generated in tbody tag, to generate in thead tag:
GridView1.UseAccessibleHeader = true;
GridView1.HeaderRow.TableSection = TableRowSection.TableHeader;
To define data-* attribute in header cells, put following code after binding:
TableCellCollection cells = GridView1.HeaderRow.Cells;
cells[0].Attributes.Add("data-class", "expand");
cells[2].Attributes.Add("data-hide", "phone,tablet");
cells[3].Attributes.Add("data-hide", "phone,tablet");
cells[4].Attributes.Add("data-hide", "phone");
We define data-hide attribute to hide the column for different dimensions. Email and Address will be hidden in tablet view and Email,Address, Contact will be hidden in phone view. On expanding, the hidden data are displayed in row by row.
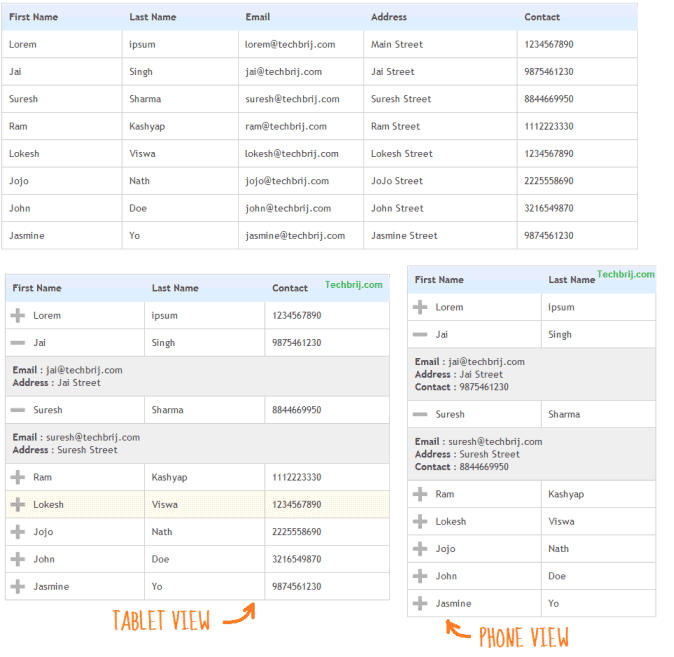
Here is the rendered html of gridview control:
<table cellspacing="0" style="border-collapse:collapse;" id="GridView1" class="footable footable-loaded default">
<thead>
<tr>
<th scope="col" data-class="expand">First Name</th><th scope="col">Last Name</th>
<th scope="col" data-hide="phone,tablet" style="display: table-cell;">Email</th>
<th scope="col" data-hide="phone,tablet" style="display: table-cell;">Address</th>
<th scope="col" data-hide="phone" style="display: table-cell;">Contact</th>
</tr>
</thead><tbody>
<tr class="">
<td class="expand">Lorem</td>
<td>ipsum</td>
<td style="display: table-cell;">[email protected]</td>
<td style="display: table-cell;">
<span id="GridView1_Label1_0">Main Street</span>
</td>
<td style="display: table-cell;">1234567890</td>
</tr>
....
</tbody>
</table>
Hope, you enjoy it.