In this tutorial series, we are going to build a simple Hello World Dapp (decentralized application) on Ethereum with Solidity, Truffle & Web3 tech stack. After this, we will deploy it to test network (Infura and Heroku). This dapp will simply store and retrieve user input text on Ethereum.
In part one, we’ll write a smart contract in Solidity using Remix. Let’s build a simple ‘Hello World’ application (The best way to learn new technologies). It is assumed you know the basics of Blockchain and its terminology. Here is quick intro of some terms:
Smart contracts: these are self-executing contracts with the terms of the agreement between buyer and seller which is directly written into lines of code.
Solidity: It is programming language to code the actual smart contracts. The syntax is very similar to JavaScript.
Remix: an online IDE, the most convenient way to develop contracts. It makes writing and debugging Solidity very easy. For this blog post, we will be using it.
Solidity
Before start to code, let's go through basic structure of solidity:
Variable types: bool, integer (int8-int256/uint8-uint256), address, bytes, string, hex and enum
Contract structure:
pragma contract ^5.0.0
contract ContractName{
<variable declaration>
<mappings>
<constructor>
<functions>
<modifiers>
}
Function syntax:
function FunctionName(Arguments...) <visibility> <state mutablitiy> returns (<return types>)
Visibility:
public — all
private — only this contract
internal — only this contract and contracts deriving from it
external — Cannot be accessed internally, only externally
State mutablitiy:
view - not to modify the state
pure - not to read from or modify the state
payable- to receive ether
Code
Open Remix IDE
pragma solidity ^0.5.0;
contract HelloWorld {
string defaultName;
constructor() public{
defaultName = 'World';
}
function getMessage() public view returns(string memory){
return concat("Hello " , defaultName);
}
}
It is straight app which returns 'Hello World'. You cannot use + operator to concat strings like in other programming languages so the following concat function is used
function concat(string memory _base, string memory _value) internal pure returns (string memory) {
bytes memory _baseBytes = bytes(_base);
bytes memory _valueBytes = bytes(_value);
string memory _tmpValue = new string(_baseBytes.length + _valueBytes.length);
bytes memory _newValue = bytes(_tmpValue);
uint i;
uint j;
for(i=0; i<_baseBytes.length; i++) {
_newValue[j++] = _baseBytes[i];
}
for(i=0; i<_valueBytes.length; i++) {
_newValue[j++] = _valueBytes[i];
}
return string(_newValue);
}
To understand data store functionality, let's make it dynamic and allow user to set name
mapping (address => string) public accounts;
function setName(string memory name) public returns(bool success){
require(bytes(name).length > 0);
accounts[msg.sender] = name;
return true;
}
mapping is like hashmap in other programming language which has collection of key value pairs. Here type of key, value are address and string respectively.
Now, let's update getMessage method to return user defined name
function getMessage() public view returns(string memory){
string memory name = bytes(accounts[msg.sender]).length > 0 ? accounts[msg.sender] : defaultName;
return concat("Hello " , name);
}
The complete code looks like below:
pragma solidity ^0.5.0;
contract HelloWorld {
string defaultName;
mapping (address => string) public accounts;
constructor() public{
defaultName = 'World';
}
function getMessage() public view returns(string memory){
string memory name = bytes(accounts[msg.sender]).length > 0 ? accounts[msg.sender] : defaultName;
return concat("Hello " , name);
}
function setName(string memory name) public returns(bool success){
require(bytes(name).length > 0);
accounts[msg.sender] = name;
return true;
}
function concat(string memory _base, string memory _value) internal pure returns (string memory) {
bytes memory _baseBytes = bytes(_base);
bytes memory _valueBytes = bytes(_value);
string memory _tmpValue = new string(_baseBytes.length + _valueBytes.length);
bytes memory _newValue = bytes(_tmpValue);
uint i;
uint j;
for(i=0; i<_baseBytes.length; i++) {
_newValue[j++] = _baseBytes[i];
}
for(i=0; i<_valueBytes.length; i++) {
_newValue[j++] = _valueBytes[i];
}
return string(_newValue);
}
}
Remix
In the right hand side, compile section, select 0.5.0 version and click "Start to compile" button. On success, you will get Hello World option.
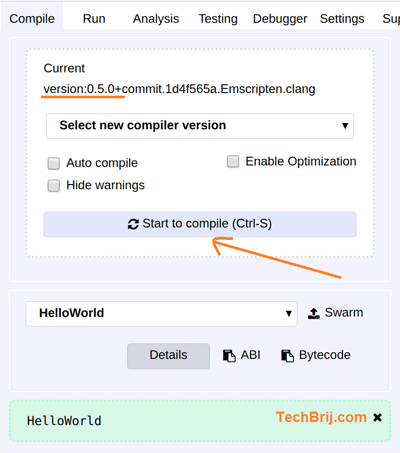
In Run tab, select Javascript VM as environment and click Deploy button. You will get "Hello World" in Deployed Contracts section.
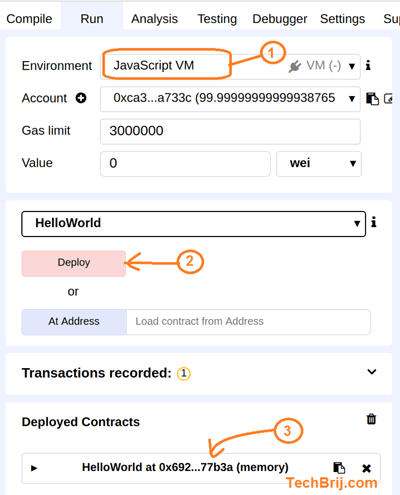
By default, on getMessage click, you will get "Hello World" response.
If you enter name and click setName then on getMessage click you will get Hello + user entered name.
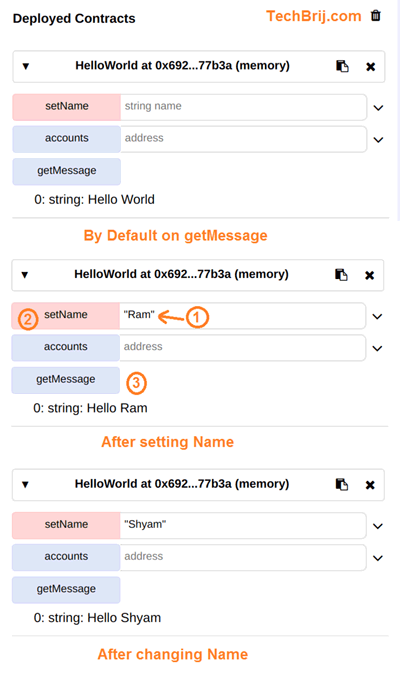
If you change account whose name is not set yet, you will get "Hello World" response.
Conclusion
In this blog post, we saw Solidity coding structure, created Hello World smart contract, went through Remix environment and tested our first contract in it. In next tutorial, we will set up our environment to start coding with Solidity on local machine.
Hope, It helps. Happy Blockchain !!