In this article, we will implement to save current geo-location(latitude, longitude) of user in Sql Server Geography data type column using Entity framework and ASP.NET Web Form.
Environment: VS2012, .NET Framework 4.5, ASP.NET Empty Website template, C#, SQL Server v11.0, Entity Framework 5.0 Database First approach.
Database Structure:
Consider the following table "PlaceInfo" in which Geolocation column (Geography data type) will be used to store current location of user.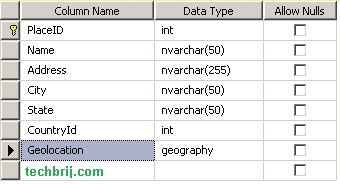
Steps:
1. Create New "ASP.NET Empty Website" project
2. Solution Explorer > Right click on project > Add New Item > ADO.NET Enitity Data Model, select database/tables, Enter proper information > Finish
3. Add New Webform > Default.aspx
4. Add jQuery reference in head tag
<script src="http://ajax.aspnetcdn.com/ajax/jquery/jquery-1.9.0.js"></script>
5. Here is the code of form
<form id="form1" runat="server">
<div class="form">
<p>
<asp:Label ID="Label1" runat="server" Text="Place Name" AssociatedControlID="txtName"></asp:Label>
<asp:TextBox ID="txtName" runat="server"></asp:TextBox>
</p>
<p>
<asp:Label ID="Label2" runat="server" Text="Address" AssociatedControlID="txtAddress"></asp:Label>
<asp:TextBox ID="txtAddress" runat="server"></asp:TextBox>
</p>
<p>
<asp:Label ID="Label3" runat="server" Text="City" AssociatedControlID="txtCity"></asp:Label>
<asp:TextBox ID="txtCity" runat="server"></asp:TextBox>
</p>
<p>
<asp:Label ID="Label4" runat="server" Text="State" AssociatedControlID="txtState"></asp:Label>
<asp:TextBox ID="txtState" runat="server"></asp:TextBox>
</p>
<p>
<asp:Label ID="Label5" runat="server" Text="Country" AssociatedControlID="txtState"></asp:Label>
<asp:DropDownList ID="ddlCountry" runat="server">
<asp:ListItem Value="1">India</asp:ListItem>
<asp:ListItem Value="2">US</asp:ListItem>
</asp:DropDownList>
</p>
<p>
<asp:HiddenField ID="hdnLocation" runat="server" />
</p>
<p>
<asp:Button ID="btnSubmit" runat="server" Text="Save" OnClick="btnSubmit_Click" />
</p>
<p id="message"></p>
</div>
</form>
In above code, Hidden field (hdnLocation) is used to save current geographical position.
6. Now we use HTML5 Geolocation API to get the geographical position of a user. Add following javascript code
if (navigator.geolocation) {
navigator.geolocation.getCurrentPosition(showPosition, showError);
}
else { $("#message").html("Geolocation is not supported by this browser."); }
function showPosition(position) {
var latlondata = position.coords.latitude + "," + position.coords.longitude;
var latlon = "Latitude" + position.coords.latitude + "," + "Longitude" + position.coords.longitude;
$("#message").html(latlon);
$("[id*=hdnLocation]").val(position.coords.longitude + " " + position.coords.latitude);
}
function showError(error) {
if (error.code == 1) {
$("#message").html("User denied the request for Geolocation.");
}
else if (error.code == 2) {
$("#message").html("Location information is unavailable.");
}
else if (error.code == 3) {
$("#message").html("The request to get user location timed out.");
}
else {
$("#message").html("An unknown error occurred.");
}
}
When page loads, you will get prompt to get permission to track your location in the browser. On allowing, the current latitude and longitude are stored in hidden field in "Longitide Latitude" format. For testing purpose, the values are displayed below button.
7. We use Entity framework to save data in database, On button click:
protected void btnSubmit_Click(object sender, EventArgs e)
{
//Validations code here
//...............
//To save in database
using ( var context = new SampleDBEntities()){
context.PlaceInfoes.Add (new PlaceInfo() {
Name = txtName.Text,
Address = txtAddress.Text,
City = txtCity.Text,
State = txtState.Text,
CountryId = Convert.ToInt32 (ddlCountry.SelectedValue),
Geolocation = DbGeography.FromText("POINT( " + hdnLocation.Value + ")")
});
context.SaveChanges();
}
}
In above server side code, hidden field value is assigned to Geolocation column. When user submits the form, the data are saved with geolocation of user in database.
Output:
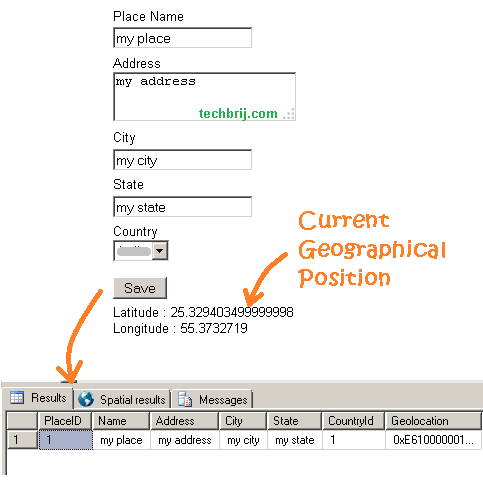
Conclusion:
We have implemented to save geographical position of user in database using HTML5 Geo-Location API and Entity framework. In next part, we will see application of Geography column to get nearest places. Stay tuned and Enjoy !!