In my last post, I spent some hours to create a stub to skip passport.authenticate method for REST API testing. So, I decided to write a separate post on the same. Before we get started, let's take a look on how auth middleware is implemented:
const router = express.Router();
router.route('/')
.get(passportManager.authenticate, projectController.get)
.post(passportManager.authenticate, projectController.add);
And passportManager has authenticate method which calls passport authenticate method as follows:
class passportManager {
...
authenticate(req, res, next){
passport.authenticate('jwt', { session: false}, (err, user, info) => {
if (err) { return next(err); }
if (!user) {
if (info.name === "TokenExpiredError") {
return res.status(401).json({ message: "Your token has expired." });
} else {
return res.status(401).json({ message: info.message });
}
}
req.user = user;
return next();
})(req, res, next);
};
}
export default new passportManager();
If you want to see the full implementation, see below post:
Token Based Authentication with Node.js, Express, Mongoose and Passport
Passport Stub
To stub passport authentication:
sinon.stub(passport,"authenticate").callsFake((strategy, options, callback) => {
callback(null, { "username": "[email protected]"}, null);
return (req,res,next)=>{};
});
Now in test methods, no need to pass token.
it("should save the project", () => {
return request.post(apiBase + "/project")
.send(newProject)
.expect(200)
.expect(res => {
res.body.success.should.be.true;
res.body.msg.should.equal("New project is created successfully.");
})
});
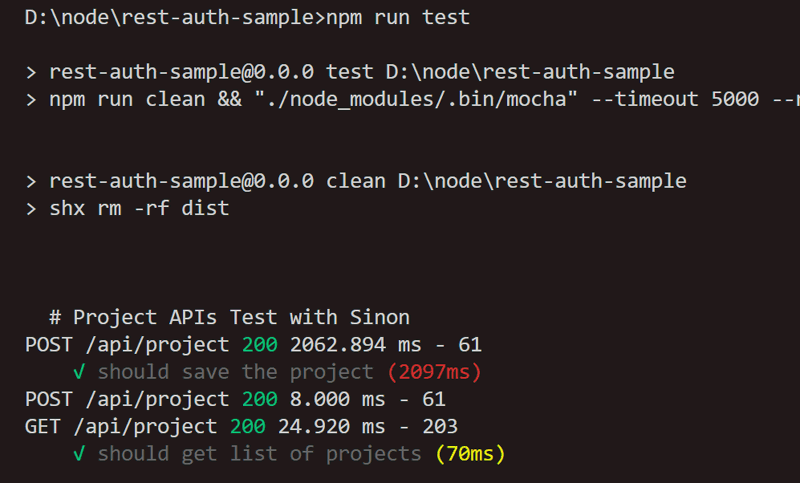
I tried different other ways to stub it but got different errors like below:
UnhandledPromiseRejectionWarning: Error: Can't set headers after they are sent.
The tricky part is what to return in stub method i.e. return (req,res,next)=>{};
Conclusion
In this post, we saw how to use Sinon stub to skip Passport authenticate. If you want to see full test methods implementation, see below:
REST API Testing using Mocha, Sinon and Chai
I hope it saves your time. Enjoy Node.js!