In this post, we will implement to get all files recursively in a directory using Node.js. Also, will restrict it to traverse directory with limited recursively depth.
Before we get started, consider the following directory structure(For simplicity, the name is according to their depth level i.e. folder 1, sub folder 1.1, sub sub folder 1.1.1...etc):
1 > 1.1 > 1.1.1> 1.1.1.1 > 1.1.1.1.1
2 > 2.1 > 2.1.1
> 2.2
Each directory has txt file with same directory name(means 1.1 will have 1.1.txt file).
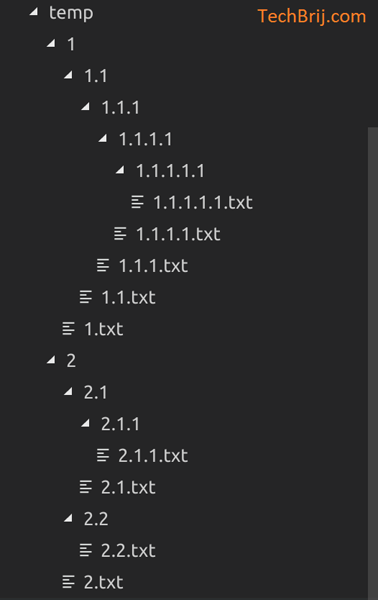
Let's start with a simple method to get all files:
function getAllFiles(dirPath){
fs.readdirSync(dirPath).forEach(function(file) {
let filepath = path.join(dirPath , file);
let stat= fs.statSync(filepath);
if (stat.isDirectory()) {
getAllFiles(filepath);
} else {
console.info(filepath+ '\n');
}
});
}
getAllFiles('./temp');
Output:
temp/1/1.1/1.1.1/1.1.1.1/1.1.1.1.1/1.1.1.1.1.txt
temp/1/1.1/1.1.1/1.1.1.1/1.1.1.1.txt
temp/1/1.1/1.1.1/1.1.1.txt
temp/1/1.1/1.1.txt
temp/1/1.txt
temp/2/2.1/2.1.1/2.1.1.txt
temp/2/2.1/2.1.txt
temp/2/2.2/2.2.txt
temp/2/2.txt
To limit recursively depth, let's add two more parameters:
function getFiles(dirPath, currentLevel, maxLevel){
if (currentLevel > maxLevel){
return;
}
else{
fs.readdirSync(dirPath).forEach(function(file) {
let filepath = path.join(dirPath , file);
let stat= fs.statSync(filepath);
if (stat.isDirectory()) {
getFiles(filepath, currentLevel+1, maxLevel);
} else {
console.info(filepath+ '\n');
}
});
}
}
maxLevel defines the maximum depth to traverse. Let's get files with max 2 levels.
getFiles('./temp',0,2);
Output:
temp/1/1.1/1.1.txt
temp/1/1.txt
temp/2/2.1/2.1.txt
temp/2/2.2/2.2.txt
temp/2/2.txt
To get files from sub-folders only, set maxLevel = 1
getFiles('./temp',0,1);
Output:
temp/1/1.txt
temp/2/2.txt
Conclusion:
In this post, we saw how to get all files from a given directory using recursion and specified max depth limit. Hope, It helps.
Enjoy Node.js!!