For a developer, Ternary operator is one of favorite thing to make the code compact and easily readable. This article explains different ways to implement Ternary operations.
In other languages like Javascript, ?: symbols are used for ternary operations.
<condition> ? <expression_on_true> : <expression_on_false>
Python 2.5+ added following syntax:
<expression_on_true> if <condition> else <expression_on_false>
expression_on_true will be evaluated if the condition is true, otherwise expression_on_false will be evaluated.
Example
n= 7
result = "Even" if n % 2 == 0 else "Odd"
print(result)
Output:
Odd
Using Tuple or Dictionary
(<expression_on_false>,<expression_on_true>)[condition]
{True:<expression_on_true>, False: <expression_on_false>}[condition]
result = ("Odd","Even")[n % 2 == 0]
print(result)
result = {False:"Odd",True:"Even"}[n % 2 == 0]
print(result)
The output is "Odd" in both cases.
Note: In this approach both expressions are evaluated regardless of the condition.
Using Lambda
In lambda, only one expression will be evaluated unlike in tuple and Dictionary
result = (lambda: "Odd", lambda: "Even")[n % 2 == 0]()
print(result)
The result will be same "Odd".
Old Techniques
Before Python 2.5, it is generally done in following way
<condition> and <expression_on_true> or <expression_on_false>
result = n % 2 == 0 and "Even" or "Odd"
Note: this won't work if expression_on_true would be False.
Common workaround is to make expression_on_true and expression_on_false lists or tuples as in the following:
result = (<condition> and [<expression_on_true>] or [<expression_on_false>])[0]
or:
result = (<condition> and (<expression_on_true>,) or (<expression_on_false>,))[0]
To understand this, consider following example:
n = 6
is_odd = n % 2 == 0 and False or True
print(is_odd)
We are expecting False but the output is True. To handle this case:
is_odd = (n % 2 == 0 and [False] or [True])[0]
print(is_odd)
or
is_odd = (n % 2 == 0 and (False,) or (True,))[0]
print(is_odd)
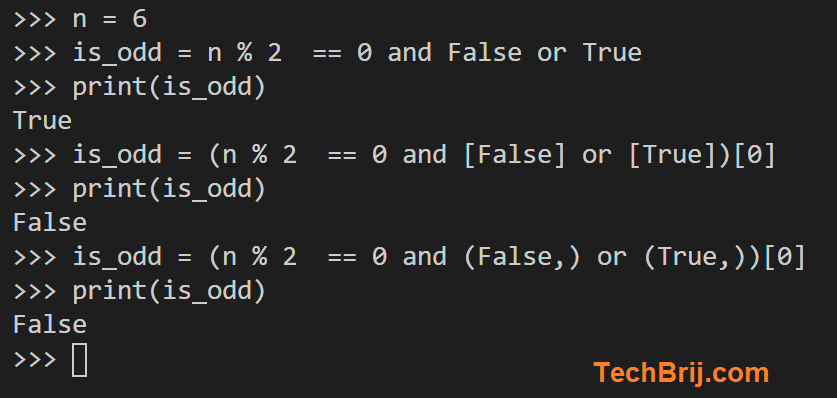
Let's shorthand some common syntax based on above approach:
expression1 if expression1 else expression2
can be written as:
expression1 or expression2
Similarly,
expression1 if expression2 else False
can be written as:
expression1 and expression2
Consider following example
default_value = 5
value = 3
setting = value if value else default_value
can be written as:
setting = value or default_value
The output will be 3. Again, you need to careful for Boolean values.
So, we saw how ternary operators shorten the code and make it more maintainable and easily readable.
Enjoy Python!!