It is annoying when your current Python test function or file is not discovered in your editor (VSCode or PyCharm) especially when all other tests are discovered but only current one is not discovered i.e. no option to run test function directly from the editor and there is no error also.
It is assumed you have already setup proper unit test configurations. The goal is to get exact reason why test method is not discovered. If there is any syntax error related to test method, then it might not be included. This post explains how to recognize and troubleshoot the issue.
Let's consider following structure of files:
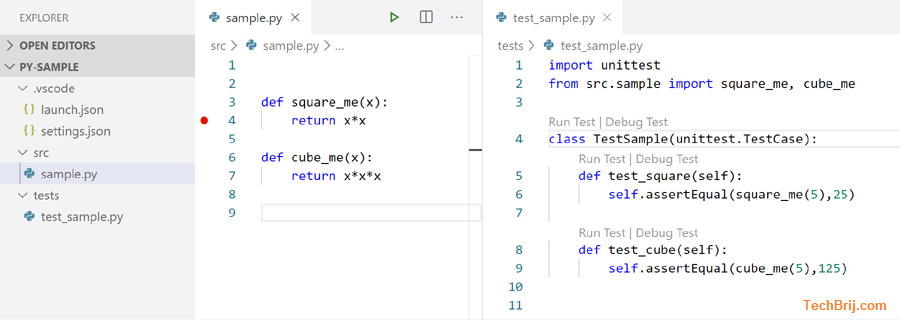
There are two folders src for source code and tests for unit test methods.
Here is the sample code in src/sample.py
def square_me(x):
return x*x
def cube_me(x):
return x*x*x
Here are the test methods in tests/test_sample.py
import unittest
from src.sample import square_me, cube_me
class TestSample(unittest.TestCase):
def test_square(self):
self.assertEqual(square_me(5),25)
def test_cube(self):
self.assertEqual(cube_me(5),125)
Let's run the test methods from terminal:
python -m unittest tests.test_sample
Output:
---------------------------------------------------------------------- Ran 2 tests in 0.000s OK
If you want to see verbose then use -v flag
python -m unittest -v tests.test_sample
Output:
test_cube (tests.test_sample.TestSample) ... ok test_square (tests.test_sample.TestSample) ... ok ---------------------------------------------------------------------- Ran 2 tests in 0.001s OK
If you want to run specific test method only, suppose to run test_cube method:
python -m unittest -v tests.test_sample.TestSample.test_cube
Output:
test_cube (tests.test_sample.TestSample) ... ok ---------------------------------------------------------------------- Ran 1 test in 0.000s OK
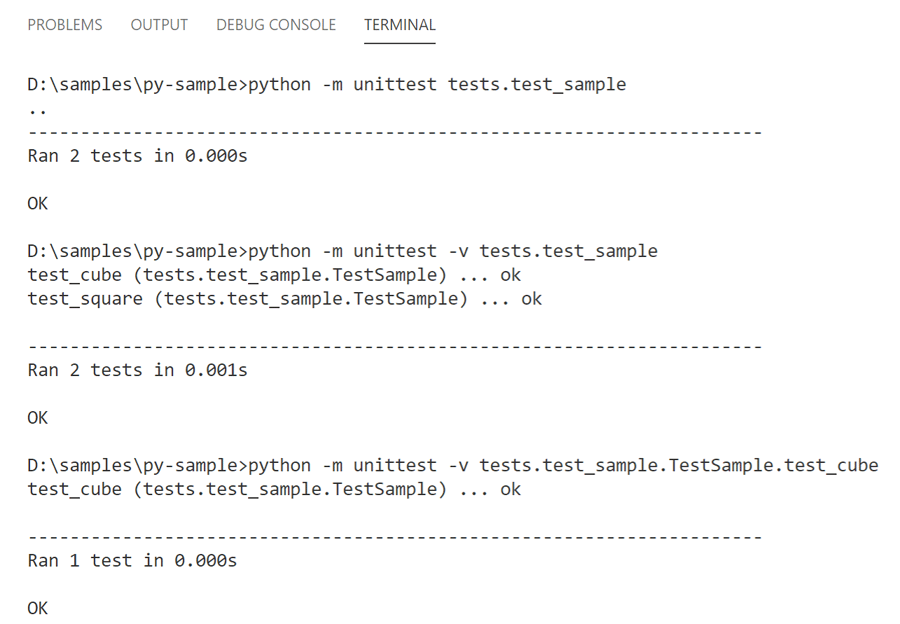
It's time to do something so that test_sample is not discovered. For this, I updated the indent in wrong order. Now, if you refresh test explorer in editor then you will not get idea why it is not discovered.
So for this run following command:
python -m unittest discover -s <directory> -p <pattern>
python -m unittest discover -s tests -p test_sample*
You will get the exact error why the test is not discovered.
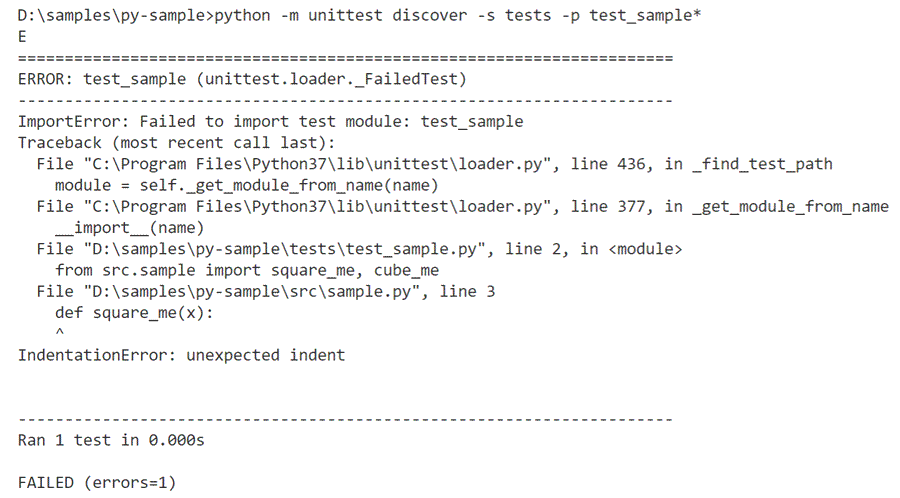
Similarly, when you run the test file directly
python -m unittest -v tests.test_sample
you will get the same error.
PyCharm and VSCode are good, but when test is not discovered automatically, these terminal commands help a lot to figure out the issue.
Enjoy Python !!