To read CSV file doesn't mean to use String.Split(). CSV files may contain commas, carriage returns, speechmarks...etc within strings. In this post, we will learn how to upload and read CSV File in ASP.NET MVC WITHOUT using Jet/ACE OLEDB provider. It is helpful when you have to deploy your code on shared hosting, Azure website or any server where ACE Database engine is not available. We will use a fast CSV Reader by Sebastien Lorien.
Read Also: Upload and Read Excel File (.xls, .xlsx) in ASP.NET MVC.
Steps:
1. Create ASP.NET MVC 5 Empty Project
2. To install CSVReader, run the following command in the Package Manager Console:
Install-Package LumenWorksCsvReader
3. Add New Controller say HomeController and add following action:
public ActionResult Upload()
{
return View();
}
4. Add View of Upload action and use following code:
@model System.Data.DataTable
@using System.Data;
<h2>Upload File</h2>
@using (Html.BeginForm("Upload", "Home", null, FormMethod.Post, new { enctype = "multipart/form-data" }))
{
@Html.AntiForgeryToken()
@Html.ValidationSummary()
<div class="form-group">
<input type="file" id="dataFile" name="upload" />
</div>
<div class="form-group">
<input type="submit" value="Upload" class="btn btn-default" />
</div>
if (Model != null)
{
<table>
<thead>
<tr>
@foreach (DataColumn col in Model.Columns)
{
<th>@col.ColumnName</th>
}
</tr>
</thead>
<tbody>
@foreach (DataRow row in Model.Rows)
{
<tr>
@foreach (DataColumn col in Model.Columns)
{
<td>@row[col.ColumnName]</td>
}
</tr>
}
</tbody>
</table>
}
}
We will read CSV file, get data in DataTable and show DataTable in View.
5. To read the submitted CSV file:
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Upload(HttpPostedFileBase upload)
{
if (ModelState.IsValid)
{
if (upload != null && upload.ContentLength > 0)
{
if (upload.FileName.EndsWith(".csv"))
{
Stream stream = upload.InputStream;
DataTable csvTable = new DataTable();
using (CsvReader csvReader =
new CsvReader(new StreamReader(stream), true))
{
csvTable.Load(csvReader);
}
return View(csvTable);
}
else
{
ModelState.AddModelError("File", "This file format is not supported");
return View();
}
}
else
{
ModelState.AddModelError("File", "Please Upload Your file");
}
}
return View();
}
It is assumed the file will have column names in first row.
Output:
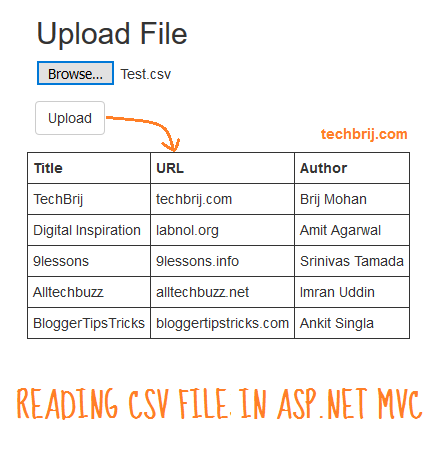
In this post, we implemented to read CSV file with CSVReader in ASP.NET MVC.
Hope It helps. Feel free to share your opinion in comment box.